Starting Out With C++ From Control Structures to Objects 8th edition by Tony Gaddis – Ebook PDF Instant Download/Delivery. 0133769399 978-0133769395
Full download Starting Out With C++ From Control Structures to Objects 8th after payment
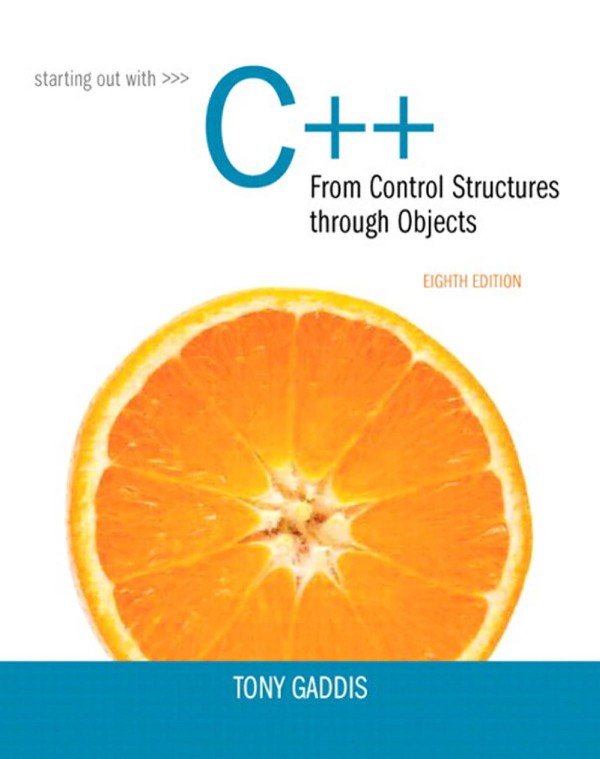
Product details:
ISBN 10: 0133769399
ISBN 13: 978-0133769395
Author: Tony Gaddis
MyProgrammingLab is not a self-paced technology and should only be purchased when required by an instructor.
This text is intended for either a one-semester accelerated introductory course or a traditional two-semester sequence covering C++ programming. It is also suitable for readers interested in a comprehensive introduction to C++ programming.
Tony Gaddis’s accessible, step-by-step presentation helps beginning students understand the important details necessary to become skilled programmers at an introductory level. Gaddis motivates the study of both programming skills and the C++ programming language by presenting all the details needed to understand the “how” and the “why”–but never losing sight of the fact that most beginners struggle with this material. His approach is both gradual and highly accessible, ensuring that students understand the logic behind developing high-quality programs.
In Starting Out with C++: From Control Structures through Objects, Gaddis covers control structures, functions, arrays, and pointers before objects and classes. As with all Gaddis texts, clear and easy-to-read code listings, concise and practical real-world examples, and an abundance of exercises appear in every chapter.
MyProgrammingLab for Starting Out with C++ is a total learning package. MyProgrammingLab is an online homework, tutorial, and assessment program that truly engages students in learning. It helps students better prepare for class, quizzes, and exams—resulting in better performance in the course—and provides educators a dynamic set of tools for gauging individual and class progress.
Teaching and Learning Experience
This program presents a better teaching and learning experience–for you and your students. It will help:
- Personalize Learning with MyProgrammingLab: Through the power of practice and immediate personalized feedback, MyProgrammingLab helps students fully grasp the logic, semantics, and syntax of programming.
- Enhance Learning with the Gaddis Approach: Gaddis’s accessible approach features clear and easy-to-read code listings, concise real-world examples, and exercises in every chapter.
- Keep Your Course Current: This edition introduces many of the new C++11 language features.
- Support Instructors and Students: Student and instructor resources are available to expand on the topics presented in the text.
Starting Out With C++ From Control Structures to Objects 8th Table of contents:
Chapter 1: Introduction to Computers and Programming
- Introduction
- Computer Systems and Software
- Overview of Programming Languages
- Introduction to C++
- Writing and Running a C++ Program
- Programming with C++: Syntax and Structure
- Understanding and Using the Console
- Programming Style and Documentation
- Summary
- Key Terms
Chapter 2: Introduction to C++
- What Is C++?
- The Structure of a C++ Program
- Variables and Data Types
- Input and Output with cin and cout
- Arithmetic Operators
- Named Constants
- Assignment and Expressions
- Type Conversion
- C++ Syntax and Style Guidelines
- Debugging and Program Development
- Summary
- Key Terms
Chapter 3: Control Structures
- Introduction to Control Structures
- If Statements
- If-Else Statements
- Nested If-Else Statements
- Switch Statements
- Logical Operators
- Relational Operators
- The Conditional Operator
- Loops: while, for, do-while
- Nested Loops
- The break and continue Statements
- Summary
- Key Terms
Chapter 4: Functions
- Introduction to Functions
- Function Definitions and Declarations
- Function Prototypes
- Passing Arguments to Functions
- Returning Values from Functions
- Function Overloading
- Default Arguments
- Scope and Lifetime of Variables
- Recursion
- Modular Program Design
- Summary
- Key Terms
Chapter 5: Arrays
- Introduction to Arrays
- Defining and Initializing Arrays
- Accessing Array Elements
- Processing Arrays
- Multidimensional Arrays
- Arrays and Functions
- Sorting Arrays
- Searching Arrays
- Summary
- Key Terms
Chapter 6: Pointers
- Introduction to Pointers
- Declaring Pointers
- The Address-of and Dereference Operators
- Pointer Arithmetic
- Arrays and Pointers
- Pointers and Functions
- Dynamic Memory Allocation
- Summary
- Key Terms
Chapter 7: Object-Oriented Programming
- Introduction to Object-Oriented Programming
- Classes and Objects
- Member Functions and Data Members
- Constructors and Destructors
- The this Pointer
- Overloading Operators
- Friend Functions
- Passing Objects to Functions
- Returning Objects from Functions
- Encapsulation, Inheritance, and Polymorphism
- Summary
- Key Terms
Chapter 8: Classes and Objects
- Introduction to Classes and Objects
- Creating and Using Classes
- Member Variables and Member Functions
- Constructors and Destructors
- Accessing Class Members
- Using Objects in Functions
- Static Members
- Operator Overloading
- Summary
- Key Terms
Chapter 9: Inheritance
- Introduction to Inheritance
- The Basics of Inheritance
- Base and Derived Classes
- Constructor and Destructor Inheritance
- Method Overriding and Virtual Functions
- Abstract Classes
- Multiple Inheritance
- Summary
- Key Terms
Chapter 10: Polymorphism
- Introduction to Polymorphism
- Function Overloading
- Operator Overloading
- Virtual Functions and Dynamic Binding
- Base and Derived Class Polymorphism
- Using Pointers and References with Polymorphism
- Summary
- Key Terms
Chapter 11: Templates
- Introduction to Templates
- Function Templates
- Class Templates
- Template Specialization
- Template Classes and Functions
- Summary
- Key Terms
Chapter 12: Exception Handling
- Introduction to Exception Handling
- Try, Catch, and Throw
- Handling Multiple Exceptions
- Creating Your Own Exceptions
- Exception Safety and Best Practices
- Summary
- Key Terms
Chapter 13: File Handling
- Introduction to File Handling
- File Streams
- Reading and Writing Files
- File Input and Output (I/O) with fstream
- Opening and Closing Files
- File Pointers
- Random Access Files
- File Handling Errors
- Summary
- Key Terms
Chapter 14: Standard Template Library (STL)
- Introduction to the STL
- The STL Container Classes
- Iterators in STL
- Algorithms in STL
- Function Objects in STL
- Using STL in C++
- Summary
- Key Terms
Chapter 15: Advanced Topics (Optional)
- Introduction to Advanced Topics
- Using Recursion in More Complex Problems
- Multithreading Basics
- More on Dynamic Memory Allocation
- Using Advanced C++ Libraries
- Summary
- Key Terms
Appendix A: Operators
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Assignment Operators
- Bitwise Operators
- Miscellaneous Operators
Appendix B: ASCII Table
Appendix C: Answers to Self-Check Exercises
Index
People also search for Starting Out With C++ From Control Structures to Objects 8th:
starting out with c++ from control structures to objects pdf
starting out with c++ from control structures through objects pdf
starting out with c++ from control structures to objects answers
starting out with c++ from control structures to objects
starting out with c++ from control structures to objects reddit