Pro TBB C++ Parallel Programming with Threading Building Blocks 1st edition by Michael Voss, Rafael Asenjo, James Reinders – Ebook PDF Instant Download/Delivery. 1484243978 978-1484243978
Full download Pro TBB C++ Parallel Programming with Threading Building Blocks 1st edition after payment
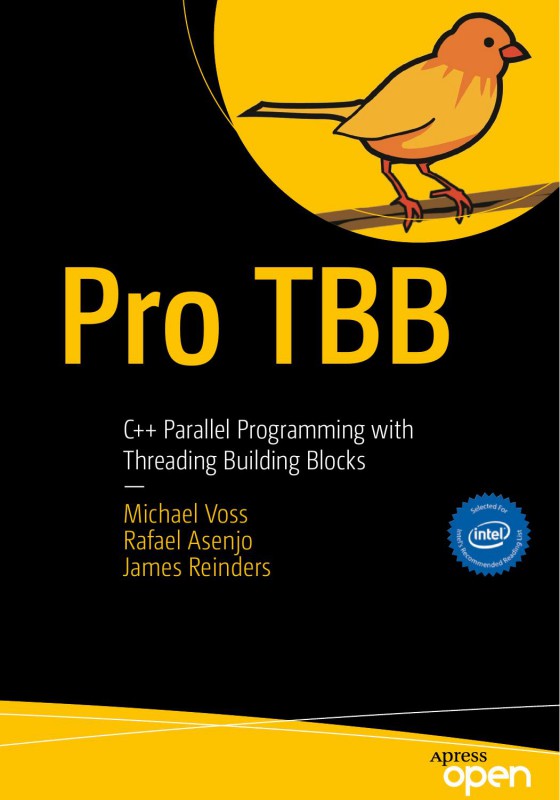
Product details:
ISBN 10: 1484243978
ISBN 13: 978-1484243978
Author: Michael Voss, Rafael Asenjo, James Reinders
This open access book is a modern guide for all C++ programmers to learn Threading Building Blocks (TBB). Written by TBB and parallel programming experts, this book reflects their collective decades of experience in developing and teaching parallel programming with TBB, offering their insights in an approachable manner. Throughout the book the authors present numerous examples and best practices to help you become an effective TBB programmer and leverage the power of parallel systems.
Pro TBB starts with the basics, explaining parallel algorithms and C++’s built-in standard template library for parallelism. You’ll learn the key concepts of managing memory, working with data structures and how to handle typical issues with synchronization. Later chapters apply these ideas to complex systems to explain performance tradeoffs, mapping common parallel patterns, controlling threads and overhead, and extending TBB to program heterogeneous systems or system-on-chips.
What You’ll Learn
- Use Threading Building Blocks to produce code that is portable, simple, scalable, and more understandable
- Review best practices for parallelizing computationally intensive tasks in your applications
- Integrate TBB with other threading packages
- Create scalable, high performance data-parallel programs
- Work with generic programming to write efficient algorithms
Who This Book Is For
C++ programmers learning to run applications on multicore systems, as well as C or C++ programmers without much experience with templates. No previous experience with parallel programming or multicore processors is required.
Pro TBB C++ Parallel Programming with Threading Building Blocks 1st Table of contents:
Part I: Getting Started with Threading Building Blocks
Chapter 1: Introduction to Parallel Programming in C++
-
Basics of parallelism and concurrency
-
Challenges in parallel programming
-
The evolution of parallel programming models
Chapter 2: Understanding Intel Threading Building Blocks (TBB)
-
Overview of TBB and its components
-
Benefits of TBB in C++ development
-
Setting up the TBB environment and tools
Part II: Core TBB Concepts and Techniques
Chapter 3: Task-Based Parallelism
-
Introduction to task parallelism
-
How TBB uses tasks for parallel execution
-
Writing efficient task-based code with TBB
Chapter 4: Parallel Algorithms in TBB
-
Overview of the parallel algorithms library
-
How to use parallel_for, parallel_reduce, and other algorithms
-
Optimizing algorithms for different hardware architectures
Chapter 5: Concurrent Containers
-
Overview of TBB’s concurrent containers (e.g., concurrent_vector, concurrent_hash_map)
-
Safe usage in multi-threaded environments
-
Practical examples with concurrent containers
Part III: Advanced TBB Features
Chapter 6: Flow Graphs for Complex Parallelism
-
Introduction to TBB Flow Graphs
-
Use cases of flow graphs in parallelism
-
Building and executing flow graphs
Chapter 7: Memory Management with TBB
-
Memory allocation and management in TBB
-
Optimizing memory for parallel workloads
-
How TBB handles memory contention
Chapter 8: Scalability and Load Balancing
-
Ensuring scalability in parallel applications
-
Load balancing techniques with TBB
-
Performance tuning and profiling with TBB
Part IV: Practical Applications and Case Studies
Chapter 9: Parallelism in Real-World Applications
-
Parallelizing computational tasks and simulations
-
Using TBB in image processing and machine learning
-
Case study: Optimizing a real-time system with TBB
Chapter 10: Debugging and Profiling Parallel Code
-
Tools for debugging parallel C++ code
-
Profiling parallel applications to find bottlenecks
-
Best practices for debugging TBB-based applications
Part V: Future Directions and Conclusion
Chapter 11: The Future of Parallel Programming
-
Upcoming trends in parallel programming
-
The role of TBB in modern C++ development
-
Integrating TBB with other libraries and frameworks
Appendices
-
A. Setting Up the Development Environment
-
B. TBB API Reference
-
C. Further Reading and Resources
-
D. Index
People also search for Pro TBB C++ Parallel Programming with Threading Building Blocks 1st:
pro tbb c++ parallel programming with threading building blocks pdf
pro tbb pdf
intel tbb parallel_for
pro tbb
tbb parallel_for example