Developing Graphics Frameworks with Python and OpenGL 1st edition by Lee Stemkoski, Michael Pascale – Ebook PDF Instant Download/Delivery. 0367721805 978-0367721800
Full download Developing Graphics Frameworks with Python and OpenGL 1st edition after payment
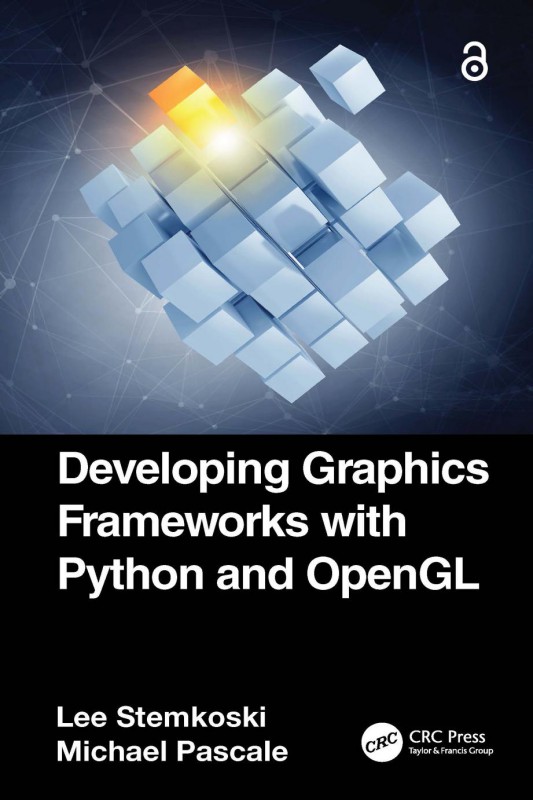
Product details:
ISBN 10: 0367721805
ISBN 13: 978-0367721800
Author: Lee Stemkoski, Michael Pascale
Developing Graphics Frameworks with Python and OpenGL shows you how to create software for rendering complete three-dimensional scenes. The authors explain the foundational theoretical concepts as well as the practical programming techniques that will enable you to create your own animated and interactive computer-generated worlds.
You will learn how to combine the power of OpenGL, the most widely adopted cross-platform API for GPU programming, with the accessibility and versatility of the Python programming language. Topics you will explore include generating geometric shapes, transforming objects with matrices, applying image-based textures to surfaces, and lighting your scene. Advanced sections explain how to implement procedurally generated textures, postprocessing effects, and shadow mapping. In addition to the sophisticated graphics framework you will develop throughout this book, with the foundational knowledge you will gain, you will be able to adapt and extend the framework to achieve even more spectacular graphical results.
Developing Graphics Frameworks with Python and OpenGL 1st Table of contents:
Part I: Getting Started with Python and OpenGL
Chapter 1: Introduction to Graphics Programming
-
Basics of computer graphics
-
The role of graphics APIs (OpenGL, Vulkan, DirectX)
-
Setting up Python for graphics programming
Chapter 2: Introduction to OpenGL
-
OpenGL concepts and pipeline overview
-
The OpenGL context and the rendering loop
-
Working with OpenGL in Python using PyOpenGL
Chapter 3: Setting Up Your Development Environment
-
Installing Python, PyOpenGL, and dependencies
-
Introduction to useful Python libraries for graphics (Pygame, NumPy, etc.)
-
Configuring a simple OpenGL window using Python
Part II: Building Basic Graphics Frameworks
Chapter 4: Drawing Basic Shapes with OpenGL
-
Understanding vertices, buffers, and shaders
-
Drawing 2D shapes (lines, rectangles, circles)
-
Simple 3D shapes (cubes, pyramids)
Chapter 5: Working with Textures
-
Introduction to textures in OpenGL
-
Loading and mapping textures onto objects
-
Applying texture filtering and transformations
Chapter 6: Implementing Lighting and Shading
-
Basic lighting models in OpenGL (ambient, diffuse, specular)
-
Writing shaders in GLSL (OpenGL Shading Language)
-
Adding dynamic lighting to 3D objects
Part III: Advanced Graphics Programming
Chapter 7: Implementing 3D Transformations
-
Scaling, rotating, and translating objects
-
Understanding the model-view and projection matrices
-
Using transformation libraries in Python
Chapter 8: Camera and Viewport Controls
-
Creating a camera class to control viewpoint
-
Implementing user input for navigation (keyboard/mouse)
-
Perspective vs orthographic projections
Chapter 9: Creating an OpenGL Framework
-
Organizing code for reusable graphics frameworks
-
Structuring a graphics engine with Python and OpenGL
-
Efficient resource management (buffers, shaders, textures)
Part IV: Creating Applications and Final Projects
Chapter 10: Animation and Interaction
-
Keyframe animation and real-time object movement
-
Responding to user input in a 3D scene
-
Collision detection and physics basics
Chapter 11: Optimizing Your OpenGL Code
-
Reducing draw calls and improving performance
-
Using OpenGL extensions and advanced techniques
-
Profiling and debugging OpenGL applications
Chapter 12: Developing a Simple Game or Visualization
-
Building a basic interactive game or graphical application
-
Integrating GUI elements for interaction
-
Final project: Full graphics application using Python and OpenGL
Part V: Future Directions
Chapter 13: The Future of Graphics Programming
-
Emerging trends in graphics technology (ray tracing, AI in graphics)
-
Beyond OpenGL: Vulkan and modern graphics APIs
-
Next steps for mastering graphics programming
Appendices
-
A. Python Resources for Graphics Programming
-
B. OpenGL Reference Guide
-
C. Further Reading and References
-
D. Index
People also search for Developing Graphics Frameworks with Python and OpenGL 1st:
developing graphics frameworks with python and opengl pdf
developing graphics frameworks with python and opengl source code
developing graphics frameworks with python and opengl code
computer graphics programming in opengl with c++ github
is gimp good for graphic design