Data Structures and Problem Solving Using C++ 2nd edition by MALIK – Ebook PDF Instant Download/Delivery. 0324782012 978-0324782011
Full download Data Structures and Problem Solving Using C++ 2nd edition after payment
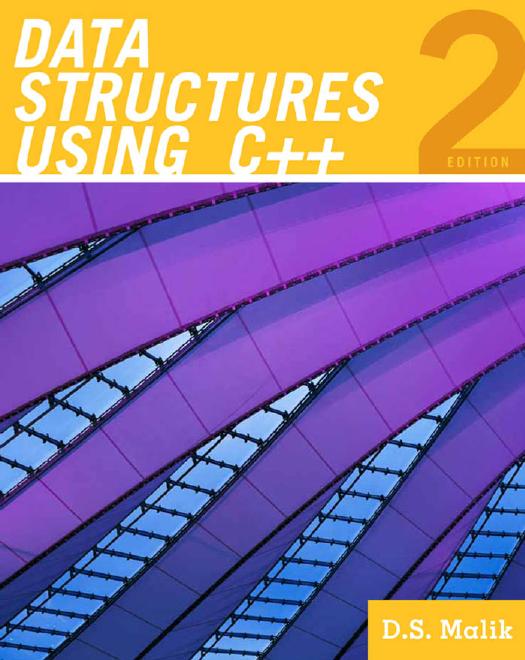
Product details:
ISBN 10: 0324782012
ISBN 13: 978-0324782011
Author: MALIK
Now in its second edition, D.S. Malik brings his proven approach to C++ programming to the CS2 course. Clearly written with the student in mind, this text focuses on Data Structures and includes advanced topics in C++ such as Linked Lists and the Standard Template Library (STL). The text features abundant visual diagrams, examples, and extended Programming Examples, all of which serve to illuminate difficult concepts. Complete programming code and clear display of syntax, explanation, and example are used throughout the text, and each chapter concludes with a robust exercise set.
Data Structures and Problem Solving Using C++ 2nd Table of contents:
Part 1: Introduction to Data Structures
Chapter 1: Introduction to Algorithms and Data Structures
1.1. Introduction to Algorithms
1.2. Performance of Algorithms
1.3. Problem Solving with Algorithms
1.4. Abstract Data Types
1.5. Introduction to C++
1.6. Problem Solving: An Overview
Chapter 2: Arrays
2.1. Introduction to Arrays
2.2. One-Dimensional Arrays
2.3. Two-Dimensional Arrays
2.4. Multidimensional Arrays
2.5. Sparse Matrices
2.6. Array-based Lists
2.7. Searching and Sorting
Part 2: Linked Lists
Chapter 3: Linked Lists
3.1. Introduction to Linked Lists
3.2. Singly Linked Lists
3.3. Doubly Linked Lists
3.4. Circular Linked Lists
3.5. Linked Lists and Recursion
3.6. Implementation of Linked Lists in C++
3.7. Linked List Operations
Chapter 4: Stacks and Queues
4.1. Stacks: Introduction and Implementation
4.2. Queue: Introduction and Implementation
4.3. Circular Queue
4.4. Applications of Stacks and Queues
4.5. Multiple Stacks and Multiple Queues
Part 3: Trees
Chapter 5: Binary Trees
5.1. Introduction to Trees
5.2. Binary Tree Structure
5.3. Traversing a Binary Tree
5.4. Binary Tree Applications
5.5. Binary Search Trees
5.6. Searching and Insertion in Binary Trees
5.7. Tree Deletion
Chapter 6: Balanced Trees
6.1. AVL Trees
6.2. Insertion and Deletion in AVL Trees
6.3. Red-Black Trees
6.4. Insertion and Deletion in Red-Black Trees
6.5. Tree Balancing and Rotations
Chapter 7: Priority Queues and Heaps
7.1. Introduction to Priority Queues
7.2. Binary Heap
7.3. Implementing Priority Queues with Heaps
7.4. Heap Sort
7.5. Applications of Heaps
Part 4: Hashing and Advanced Topics
Chapter 8: Hashing
8.1. Introduction to Hashing
8.2. Hash Functions
8.3. Collision Resolution Techniques
8.4. Hash Tables
8.5. Rehashing
8.6. Applications of Hashing
Chapter 9: Graphs
9.1. Introduction to Graphs
9.2. Representation of Graphs
9.3. Depth-First Search (DFS)
9.4. Breadth-First Search (BFS)
9.5. Shortest Path Algorithms
9.6. Minimum Spanning Trees
Chapter 10: Advanced Data Structures
10.1. Disjoint Sets
10.2. Splay Trees
10.3. B-Trees
10.4. Tries
10.5. Bloom Filters
Part 5: Problem Solving with Data Structures
Chapter 11: Algorithmic Problem Solving
11.1. Problem-Solving Strategy
11.2. Divide and Conquer
11.3. Dynamic Programming
11.4. Greedy Algorithms
11.5. Backtracking
11.6. Branch and Bound
Chapter 12: Sorting and Searching
12.1. Introduction to Sorting
12.2. Bubble Sort
12.3. Selection Sort
12.4. Insertion Sort
12.5. Merge Sort
12.6. Quick Sort
12.7. Heap Sort
12.8. Linear Search and Binary Search
Appendices
-
A. Complexity of Algorithms
-
B. C++ Reference
-
C. Answers to Selected Exercises
-
D. Index
People also search for Data Structures and Problem Solving Using C++ 2nd:
problem solving with algorithms and data structures using c++
data structures and algorithms in c++ solutions
data structures and algorithms in c++ 2nd edition solutions pdf
data structures and algorithm analysis in c++ solution manual pdf
data structure and algorithms using c++ a practical implementation