Data Structures, Algorithms, and Applications in C++ With Microsoft Compiler 2nd edition by Michael Goodrich, Roberto Tamassia, David Mount – Ebook PDF Instant Download/Delivery. B005FHM6X2 978-0470383278
Full download Data Structures, Algorithms, and Applications in C++ With Microsoft Compiler 2nd edition after payment
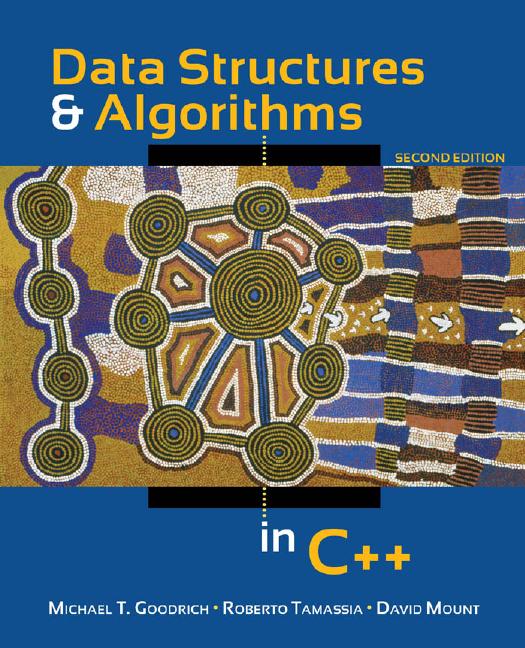
Product details:
ISBN 10: B005FHM6X2
ISBN 13: 978-0470383278
Author: Michael Goodrich, Roberto Tamassia, David Mount
This second edition of Data Structures and Algorithms in C++ is designed to provide an introduction to data structures and algorithms, including their design, analysis, and implementation. The authors offer an introduction to object-oriented design with C++ and design patterns, including the use of class inheritance and generic programming through class and function templates, and retain a consistent object-oriented viewpoint throughout the book. This is a sister book to Goodrich s Data Structures and Algorithms in Java, but uses C++ as the basis language instead of Java. This C++ version retains the same pedagogical approach and general structure as the Java version so schools that teach data structures in both C++ and Java can share the same core syllabus. In terms of curricula based on the IEEE/ACM 2001 Computing Curriculum, this book is appropriate for use in the courses CS102 (I/O/B versions), CS103 (I/O/B versions), CS111 (A version), and CS112 (A/I/O/F/H versions).
Data Structures, Algorithms, and Applications in C++ With Microsoft Compiler 2nd Table of contents:
Chapter 1: Introduction to Data Structures and Algorithms
- Data Structures and Algorithms Overview
- Introduction to the C++ Programming Language
- The Role of Algorithms in Problem Solving
- The Concept of Abstract Data Types (ADTs)
- The Importance of Efficiency in Algorithms
- The C++ Standard Template Library (STL)
Chapter 2: Arrays and Linked Lists
- Introduction to Arrays
- One-Dimensional Arrays
- Multi-Dimensional Arrays
- Introduction to Linked Lists
- Singly Linked Lists
- Doubly Linked Lists
- Circular Linked Lists
- Applications of Linked Lists
Chapter 3: Stacks
- Introduction to Stacks
- Stack Operations
- Applications of Stacks
- Stack Implementation using Arrays and Linked Lists
- Evaluation of Arithmetic Expressions
- Parsing Expressions
- Recursion and the Stack
Chapter 4: Queues
- Introduction to Queues
- Queue Operations
- Circular Queues
- Priority Queues
- Deques (Double-Ended Queues)
- Applications of Queues
- Queue Implementation
Chapter 5: Trees
- Introduction to Trees
- Binary Trees
- Binary Search Trees (BST)
- Tree Traversal Techniques
- AVL Trees (Self-Balancing Binary Search Trees)
- B-Trees and Red-Black Trees
- Applications of Trees
- Tree Algorithms and Their Analysis
Chapter 6: Heaps
- Introduction to Heaps
- Binary Heaps
- Heap Operations
- Priority Queues using Heaps
- Heap Sort Algorithm
- Applications of Heaps
Chapter 7: Hashing
- Introduction to Hashing
- Hash Functions
- Collision Resolution Strategies
- Open Addressing
- Chaining
- Applications of Hashing
- Hash Tables and Hash Maps
Chapter 8: Searching Algorithms
- Introduction to Searching
- Linear Search
- Binary Search
- Search Trees and Balanced Trees
- Applications of Searching Algorithms
- Hashing and its Role in Searching
Chapter 9: Sorting Algorithms
- Introduction to Sorting
- Comparison-Based Sorting Algorithms
- Bubble Sort
- Insertion Sort
- Selection Sort
- Merge Sort
- Quick Sort
- Heap Sort
- Non-Comparison-Based Sorting
- Counting Sort
- Radix Sort
- Bucket Sort
- Sorting Applications and Analysis
Chapter 10: Graphs
- Introduction to Graphs
- Graph Representation: Adjacency Matrix and List
- Graph Traversals: Depth-First Search (DFS) and Breadth-First Search (BFS)
- Shortest Path Algorithms
- Dijkstra’s Algorithm
- Bellman-Ford Algorithm
- Minimum Spanning Tree
- Prim’s Algorithm
- Kruskal’s Algorithm
- Topological Sorting
- Applications of Graphs
Chapter 11: Dynamic Programming
- Introduction to Dynamic Programming
- The Greedy Method vs Dynamic Programming
- Memoization and Tabulation
- Fibonacci Sequence
- Matrix Chain Multiplication
- Longest Common Subsequence
- Knapsack Problem
- Applications of Dynamic Programming
Chapter 12: Divide and Conquer
- Introduction to Divide and Conquer
- The Divide and Conquer Paradigm
- Merge Sort
- Quick Sort
- Closest Pair of Points Problem
- Strassen’s Matrix Multiplication
- Applications of Divide and Conquer
Chapter 13: Greedy Algorithms
- Introduction to Greedy Algorithms
- The Greedy Approach
- Knapsack Problem (Greedy Solution)
- Huffman Coding
- Job Scheduling Problem
- Applications of Greedy Algorithms
Chapter 14: Complexity Analysis
- Introduction to Algorithm Analysis
- Big O Notation
- Time and Space Complexity
- Analyzing Recursive Algorithms
- Amortized Analysis
- Worst-Case, Best-Case, and Average-Case Analysis
Chapter 15: Memory Management and Storage Structures
- Introduction to Memory Management
- Stack vs. Heap Memory Allocation
- Memory Leaks and Garbage Collection
- Memory Pools
- Garbage Collection Techniques in C++
- File Handling and I/O in C++
- Data Storage Techniques: Arrays, Lists, and Trees
Chapter 16: Advanced Topics in Algorithms
- Computational Geometry
- Network Flow Algorithms
- NP-Completeness and Approximation Algorithms
- Randomized Algorithms
- Parallel Algorithms
- Applications in Modern Computing
Appendices
- Appendix A: C++ Syntax Reference
- Appendix B: Standard Template Library (STL) Overview
- Appendix C: Sample Programs and Code Listings
- Appendix D: Answers to Selected Exercises
Index
People also search for Data Structures, Algorithms, and Applications in C++ With Microsoft Compiler 2nd:
borrow data structures algorithms and applications in c++
data structures algorithms and applications in java sartaj sahni pdf
data structures algorithms and applications in java pdf
data structures algorithms and applications in java sartaj sahni
data structures algorithms and applications