ANSI Common LISP (Prentice Hall series in artificial intelligence) 1st edition by Paul Graham – Ebook PDF Instant Download/Delivery. 0133708756 978-0133708752
Full download ANSI Common LISP (Prentice Hall series in artificial intelligence) 1st edition after payment
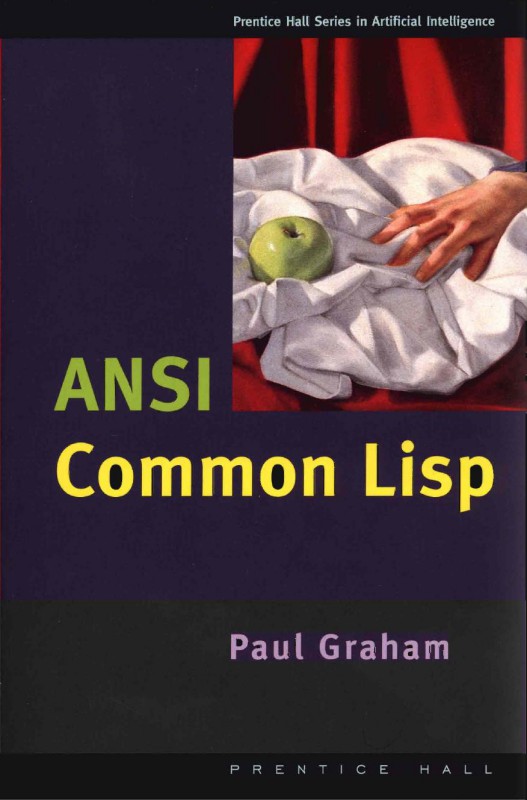
Product details:
ISBN 10: 0133708756
ISBN 13: 978-0133708752
Author: Paul Graham
For use as a core text supplement in any course covering common LISP such as Artificial Intelligence or Concepts of Programming Languages.
Teaching students new and more powerful ways of thinking about programs, this text contains a tutorial―full of examples―that explains all the essential concepts of Lisp programming, plus an up- to-date summary of ANSI Common Lisp, listing every operator in the language. Informative and fun, it gives students everything they need to start writing programs in Lisp both efficiently and effectively, and highlights such innovative Lisp features as automatic memory management, manifest typing, closures, and more.
ANSI Common LISP (Prentice Hall series in artificial intelligence) 1st Table of contents:
Preface
- Introduction to the Book
- Who This Book is For
- Structure and Approach
- Overview of Common Lisp and its Role in AI
- How to Use This Book
Part I: Introduction to Common Lisp
-
Introduction to Common Lisp
- What is Common Lisp?
- History and Evolution of Lisp
- Features and Strengths of Common Lisp
- Common Lisp vs. Other Programming Languages
- Setting Up a Lisp Environment
- Writing Your First Lisp Program
-
Basic Lisp Syntax and Semantics
- The Structure of Lisp Expressions
- Data Types in Lisp: Numbers, Strings, Lists, and Symbols
- Basic Arithmetic and Operations
- Variables and Assignment
- Functions: Defining and Calling Functions
- Special Forms:
defun
,setq
,if
, andquote
-
Control Structures and Conditional Statements
- Conditional Expressions:
if
,cond
,case
,when
, andunless
- Iteration:
loop
,do
, anddotimes
- Recursion in Lisp: A Key Paradigm
- Using
return
,go
, andblock
for Control Flow - Error Handling and Debugging with Lisp
- Conditional Expressions:
Part II: Advanced Lisp Programming
-
Working with Lists and Sequences
- List Operations:
car
,cdr
,cons
, andlist
- Higher-Order Functions:
mapcar
,reduce
,filter
- Lists vs. Arrays: Differences and Use Cases
- Sequences and Iteration:
dolist
,loop
, andmap
- Lists as Data Structures: Stacks, Queues, and Trees
- List Operations:
-
Functions and Closures
- Defining Functions with
defun
andlambda
- Closures and Lexical Scoping
- Function Specialization and Multiple Values
- Using
funcall
,apply
, andmapcar
for Function Calls - Advanced Function Techniques: Recursion, Tail-Call Optimization
- Defining Functions with
-
Macros and Code Expansion
- Understanding Macros in Lisp
- Defining Macros with
defmacro
- Code Expansion and Evaluation
- Macro-Expansion for Code Generation
- Using Macros to Simplify Code
-
Object-Oriented Programming in Common Lisp
- Introduction to Object-Oriented Programming
- The CLOS (Common Lisp Object System)
- Defining Classes and Methods in CLOS
- Generic Functions and Method Dispatch
- Inheritance and Polymorphism in CLOS
- Defining and Using CLOS Instances
Part III: Practical Lisp Programming for AI
-
Data Structures and Algorithms
- Common Data Structures in Lisp: Lists, Arrays, Hash Tables
- Searching and Sorting Algorithms
- Symbol Tables and Hashing
- Priority Queues and Balanced Trees
- Graphs and Networks in Lisp
-
Common Lisp for Artificial Intelligence
- Overview of AI Programming in Lisp
- Symbolic Computation and AI Problem Solving
- Knowledge Representation and Reasoning
- Search Algorithms in Lisp
- Implementing Heuristics and Decision Trees
-
Natural Language Processing in Lisp
- Text Processing: Parsing and Tokenization
- Regular Expressions in Lisp
- Syntax Trees and Parsing Techniques
- Implementing Simple Natural Language Processing (NLP) Tasks
- Lisp Applications in Speech Recognition and Understanding
- Machine Learning and Lisp
- Machine Learning Algorithms in Lisp: Supervised vs. Unsupervised
- Implementing Neural Networks and Genetic Algorithms
- Lisp Libraries for Machine Learning
- Optimization Techniques in AI
- Building AI Models with Lisp for Pattern Recognition
Part IV: The Advanced Features of Common Lisp
- Advanced Data Types and Structures
- Streams: Reading and Writing Files in Lisp
- CLOS Advanced Features: Multiple Inheritance, Method Combinations
- Complex Numbers, Rational Numbers, and Bit-Vector Operations
- Hash Tables and Property Lists
- Object Serialization and Persistence
- Performance and Optimization in Lisp
- Profiling and Performance Tuning
- Memory Management and Garbage Collection
- Compiler Optimization and Speed Improvements
- Using the
declare
andoptimize
Forms - Debugging and Tracing in Lisp
- Interfacing with Other Languages
- Calling C from Lisp with Foreign Function Interface (FFI)
- Interfacing with Java and Python via JNI and Other Interfaces
- Working with External Libraries in Lisp
- Integrating Lisp with Databases and Web Services
Part V: Final Thoughts
- Common Lisp in the Real World
- Common Lisp Applications in AI, Robotics, and Beyond
- The Role of Lisp in Modern Software Engineering
- Lisp vs. Other AI Languages: Python, Prolog, etc.
- Using Lisp for Rapid Prototyping and Research
- Challenges and Advantages of Using Lisp in AI
- The Future of Lisp and AI
- The Evolving Landscape of AI and Lisp’s Role
- Continuing Development of Lisp Standards
- Lisp in the Age of Machine Learning and Deep Learning
- Community, Libraries, and Resources for Lisp Programming
References
- Key Research Papers, Books, and Online Resources for Further Reading
Index
People also search for ANSI Common LISP (Prentice Hall series in artificial intelligence) 1st :
prentice hall series in artificial intelligence
a.i. artificial intelligence 2
f artificial intelligence
artificial general intelligence pdf
o’reilly artificial intelligence conference