Java: How to Program 10th edition by Harvey Deitel, Paul Deitel – Ebook PDF Instant Download/Delivery. 0133807800 978-0133807806
Full download Java: How to Program 10th edition after payment
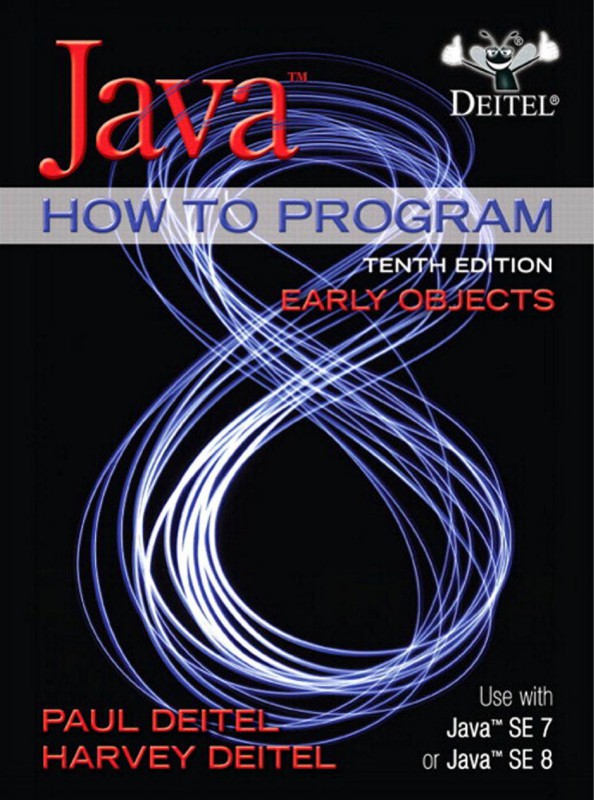
Product details:
ISBN 10: 0133807800
ISBN 13: 978-0133807806
Author: Harvey Deitel, Paul Deitel
MyProgrammingLab should only be purchased when required by an instructor.
Java How to Program (Early Objects) , Tenth Edition is intended for use in the Java programming course. It also serves as a useful reference and self-study tutorial to Java programming.
The Deitels’ groundbreaking How to Program series offers unparalleled breadth and depth of object-oriented programming concepts and intermediate-level topics for further study. Java How to Program (Early Objects), Tenth Edition, teaches programming by presenting the concepts in the context of full working programs and takes an early-objects approach(
MyProgrammingLab for Java How to Program (Early Objects) is a total learning package. MyProgrammingLab is an online homework, tutorial, and assessment program that truly engages students in learning. It helps students better prepare for class, quizzes, and exams–resulting in better performance in the course–and provides educators a dynamic set of tools for gauging individual and class progress.
Teaching and Learning Experience
This program presents a better teaching and learning experience–for you and your students.
• Personalize Learning with MyProgrammingLab: Through the power of practice and immediate personalized feedback, MyProgrammingLab helps students fully grasp the logic, semantics, and syntax of programming.
• Teach Programming with the Deitels’ Signature Live Code Approach: Java language features are introduced with thousands of lines of code in hundreds of complete working programs.
• Introduce Objects Early: Presenting objects and classes early gets students “thinking about objects” immediately and mastering these concepts more thoroughly.
• Keep Your Course Current: This edition can be used with Java SE 7 or Java SE 8, and is up-to-date with the latest technologies and advancements.
• Facilitate Learning with Outstanding Applied Pedagogy: Making a Difference exercise sets, projects, and hundreds of valuable programming tips help students apply concepts.
• Support Instructors and Students: Student and instructor resources are available to expand on the topics presented in the text.
Java: How to Program 10th Table of contents:
-
Introduction to Computers and Java
- Introduction to computer science and programming
- The Java programming language and its evolution
- Installing and setting up Java development environment
- Writing your first Java program
-
Object-Oriented Programming (OOP) Concepts
- The fundamentals of object-oriented programming
- Classes and objects
- Methods, constructors, and instance variables
- The concept of encapsulation and access modifiers
-
Control Statements
- Conditional statements (if-else, switch)
- Loops (for, while, do-while)
- Break and continue
- Nested control structures
-
Arrays
- Introduction to arrays in Java
- Single-dimensional arrays
- Multidimensional arrays
- Array manipulation and processing
-
Methods and Parameter Passing
- Method definition, parameters, and return values
- Method overloading
- Passing arguments by value
- Recursion
-
Classes and Objects
- Defining classes and objects
- Instance variables and methods
- Constructors and initialization
- Static variables and methods
-
Inheritance
- Basic inheritance and class hierarchies
- Method overriding
- The
super
keyword - Polymorphism and dynamic method dispatch
-
Exception Handling
- Introduction to exceptions
- Try-catch blocks
- Throwing exceptions
- The
finally
block - Creating custom exceptions
-
Graphical User Interface (GUI)
- Introduction to GUI programming in Java
- Using Java Swing components (JFrame, JButton, JTextField)
- Event handling and listeners
- Layout managers and GUI design principles
-
Java Collections Framework
- The Java Collections framework overview
- Lists, sets, and maps
- Iterators and the enhanced for loop
- Using ArrayList, LinkedList, HashSet, HashMap
-
File I/O and Serialization
- Reading and writing text files
- Working with file streams and readers/writers
- Object serialization and deserialization
- The
java.nio
package
-
Multithreading
- Introduction to threads and concurrency
- Creating and running threads
- Thread synchronization
- Thread lifecycle and synchronization issues
-
Lambda Expressions and Streams
- Introduction to functional programming
- Using lambda expressions in Java
- Streams API for processing collections
- Using filter, map, and reduce operations
-
JavaFX for GUI Programming
- Introduction to JavaFX for modern desktop applications
- JavaFX components (buttons, labels, text fields)
- Event handling and animations in JavaFX
- Building applications with JavaFX
-
Networking
- Introduction to network programming in Java
- Client-server model
- Using sockets for communication
- HTTP and URL processing
-
Database Access with JDBC
- Introduction to databases and JDBC
- Connecting to databases and executing SQL queries
- Handling results and managing database connections
- Prepared statements and batch processing
-
Advanced Topics
- Generic types and collections
- Reflection API
- Design patterns and best practices
- Unit testing with JUnit
-
Conclusion and Final Remarks
- Review of core concepts
- Tips for continuing Java programming and further learning
- Final exercises and projects
People also search for Java: How to Program 10th :
java how to program 11th edition github
java how to program 13th
java how to program 10th edition github
java how to program deitel ppt
java how to program 11th