Assembly Language for X86 Processors 7th edition by Kip Irvine – Ebook PDF Instant Download/Delivery. 0133769402 978-0133769401
Full download Assembly Language for X86 Processors 7th edition after payment
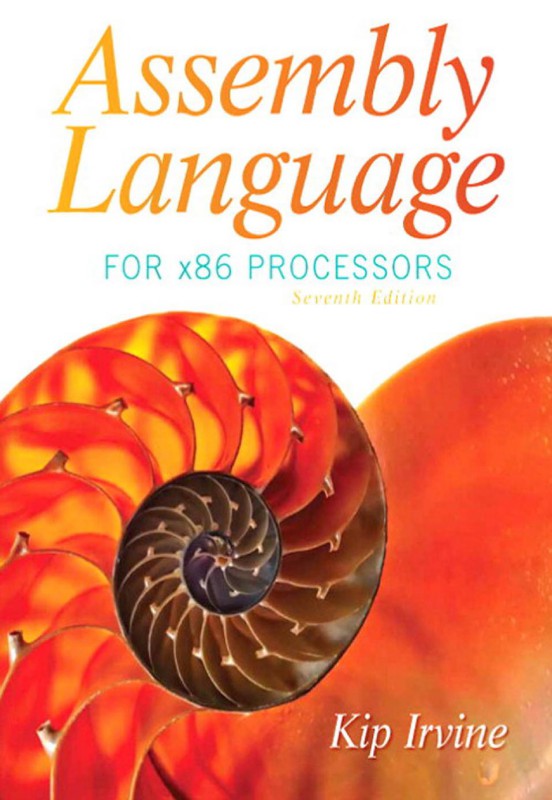
Product details:
ISBN 10: 0133769402
ISBN 13: 978-0133769401
Author: Kip Irvine
Assembly Language for x86 Processors, 7e is intended for use in undergraduate courses in assembly language programming and introductory courses in computer systems and computer architecture. This title is also suitable for embedded systems programmers and engineers, communication specialists, game programmers, and graphics programmers. Proficiency in one other programming language, preferably Java, C, or C++, is recommended.
Written specifically for 32- and 64-bit Intel/Windows platform, this complete and fullyupdated study of assembly language teaches students to write and debug programs at the machine level. This text simplifies and demystifies concepts that students need to grasp before they can go on to more advanced computer architecture and operating systems courses. Students put theory into practice through writing software at the machine level, creating a memorable experience that gives them the confidence to work in any OS/machine-oriented environment.
Assembly Language for X86 Processors 7th Table of contents:
Chapter 1: Introduction to Assembly Language
1.1. What is Assembly Language?
1.2. The x86 Processor Family
1.3. The Role of Assembly Language in Computer Systems
1.4. Overview of the 32-bit and 64-bit x86 Architectures
1.5. Assembly Language vs. High-Level Languages
1.6. The Structure of an Assembly Program
1.7. Basic Syntax and Instruction Format
Chapter 2: The x86 Processor
2.1. Processor Architecture
2.2. Registers and Their Roles
2.3. The Stack and Stack Operations
2.4. The Memory Addressing Model
2.5. The ALU and Its Operations
2.6. Instruction Execution and Fetch-Decode-Execute Cycle
2.7. Overview of the x86 Instruction Set
Chapter 3: Arithmetic and Logic Instructions
3.1. Addition, Subtraction, and Multiplication Instructions
3.2. Division Instructions
3.3. Logic Instructions (AND, OR, XOR, NOT)
3.4. Shift and Rotate Operations
3.5. Signed and Unsigned Arithmetic
3.6. Comparison and Conditional Jumps
3.7. Implementing Simple Algorithms Using Assembly
Chapter 4: Control Flow
4.1. Unconditional Branches (JMP)
4.2. Conditional Branches (JZ, JNZ, JC, etc.)
4.3. Loops and Loop Control Instructions
4.4. Procedures (Functions) and Call Stack
4.5. Using the CALL and RET Instructions
4.6. Handling Interrupts and Exceptions
4.7. Subroutine Design and Stack Frames
Chapter 5: The Stack and Memory
5.1. Introduction to the Stack
5.2. Stack Operations: PUSH, POP
5.3. The CALL and RET Instructions and the Stack
5.4. Local and Global Variables
5.5. Memory Access: Direct, Indirect, and Indexed Addressing
5.6. The Stack Frame: Pushing and Popping Parameters
5.7. Stack-Based Data Structures (Arrays, Linked Lists)
5.8. Memory Allocation and Management in Assembly
Chapter 6: Data Representation
6.1. Binary, Hexadecimal, and Decimal Number Systems
6.2. Signed and Unsigned Integers
6.3. Floating-Point Representation
6.4. Character Representation: ASCII and Unicode
6.5. Conversion between Different Data Formats
6.6. Bitwise Manipulations
6.7. Data Alignment and Padding
Chapter 7: Input and Output
7.1. Handling I/O in Assembly Language
7.2. System Calls and Interrupts for I/O
7.3. Reading from and Writing to Files
7.4. String Processing and Manipulation
7.5. Keyboard Input and Display Output
7.6. Implementing I/O in a Simple Program
7.7. Using External Libraries for I/O
Chapter 8: Assembly Language for the x86-64 Architecture
8.1. Introduction to 64-bit x86 Architecture
8.2. 64-bit Registers and Addressing Modes
8.3. The Extended Instruction Set for 64-bit Processors
8.4. Calling Conventions in x86-64
8.5. System Programming with x86-64
8.6. Converting 32-bit Programs to 64-bit
8.7. Memory Management in 64-bit Systems
Chapter 9: Optimizing Assembly Code
9.1. Introduction to Optimization Techniques
9.2. Code Size and Speed Optimization
9.3. The Role of Compiler Optimization
9.4. Using Efficient Data Structures in Assembly
9.5. Loop Unrolling and Instruction Pipelining
9.6. Minimizing Branch Instructions
9.7. Performance Testing and Profiling
9.8. Case Study: Optimizing an Assembly Program
Chapter 10: Systems Programming with Assembly
10.1. Operating System Services and System Calls
10.2. Writing a Simple Shell Program
10.3. Memory Management and Virtual Memory
10.4. Writing Device Drivers in Assembly
10.5. Multithreading and Synchronization in Assembly
10.6. Interfacing with C Programs
10.7. Debugging Assembly Programs
10.8. Writing a Simple OS Kernel in Assembly
Chapter 11: Assembly Language and the Windows Environment
11.1. The Windows Operating System and Assembly Language
11.2. Writing Windows API Functions in Assembly
11.3. Dynamic Linking and Using DLLs in Assembly
11.4. Accessing Windows System Calls
11.5. Using the Windows Debugger for Assembly Programs
11.6. Writing Windows Applications with Assembly Language
Chapter 12: Advanced Topics
12.1. The x86 Interrupt Mechanism
12.2. Writing Interrupt Service Routines (ISRs)
12.3. System-Level Programming in Assembly
12.4. Exploiting Hardware Features for Performance
12.5. Cryptography and Security with Assembly Language
12.6. The Role of Assembly Language in Embedded Systems
12.7. Future of Assembly Language Programming
Appendices
A. Instruction Set Summary
B. ASCII Character Set
C. Common Debugging Techniques
D. Sample Programs
E. Answers to Exercises
F. Index
People also search for Assembly Language for X86 Processors 7th:
kip irvine assembly language for x86 processors
assembly language for x86 processors 7th edition
assembly language for x86 processors 8th edition
assembly language for x86 processors seventh edition
assembly language for x86 processors 8th edition pdf reddit