Outlines and Highlights for Data Structures and Algorithm Analysis in C++ 3rd edition by Mark Allen Weiss – Ebook PDF Instant Download/Delivery. 032144146X 9780321441461
Full download Outlines and Highlights for Data Structures and Algorithm Analysis in C++ 3rd edition after payment
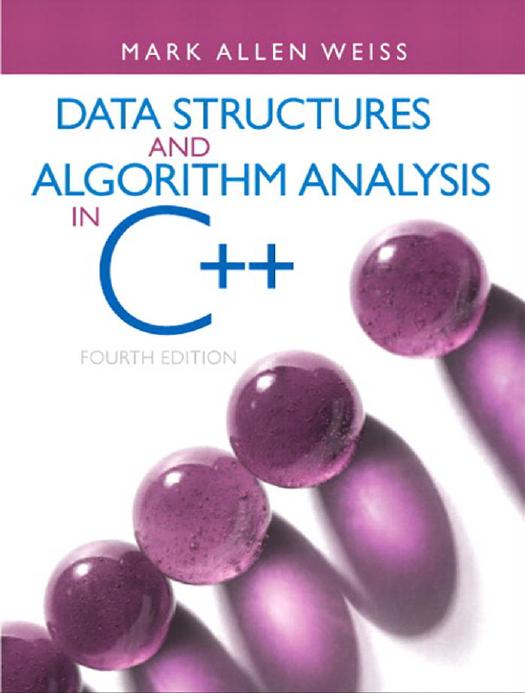
Product details:
ISBN 10: 032144146X
ISBN 13: 9780321441461
Author: Mark Allen Weiss
In this text, readers are able to look at specific problems and see how careful implementations can reduce the time constraint for large amounts of data from several years to less than a second. Class templates are used to describe generic data structures and first-class versions of vector and string classes are used. Included is an appendix on a Standard Template Library (STL). This text is for readers who want to learn good programming and algorithm analysis skills simultaneously so that they can develop such programs with the maximum amount of efficiency. Readers should have some knowledge of intermediate programming, including topics as object-based programming and recursion, and some background in discrete math.
Outlines and Highlights for Data Structures and Algorithm Analysis in C++ 3rd Table of contents:
Chapter 1: Introduction
1.1. What is an Algorithm?
1.2. The Role of Algorithms in Computing
1.3. Abstract Data Types and Data Structures
1.4. Efficiency of Algorithms
1.5. Performance Analysis and Big-O Notation
1.6. Algorithm Design Techniques
Chapter 2: Basic Data Structures
2.1. Arrays
2.2. Linked Lists
2.3. Stacks
2.4. Queues
2.5. Deques
2.6. Review of C++ Class Design
2.7. Implementation of Basic Data Structures
Chapter 3: Algorithm Analysis
3.1. Worst-Case and Average-Case Analysis
3.2. Recurrences and Recursive Algorithms
3.3. The Master Theorem for Divide and Conquer Recurrences
3.4. Solving Recurrences Using Substitution Method
3.5. Recursion and Iteration
Chapter 4: Recursion and Recursive Algorithms
4.1. Introduction to Recursion
4.2. Recursive Algorithms
4.3. Implementing Recursive Algorithms
4.4. Solving Recursion with the Divide-and-Conquer Approach
4.5. Backtracking Algorithms
4.6. Recursive Data Structures
Chapter 5: Sorting Algorithms
5.1. Bubble Sort and Insertion Sort
5.2. Selection Sort
5.3. Merge Sort
5.4. Quick Sort
5.5. Heap Sort
5.6. Counting Sort and Radix Sort
5.7. Comparison of Sorting Algorithms
5.8. Stability and Sorting Efficiency
Chapter 6: Trees
6.1. Binary Trees
6.2. Binary Search Trees
6.3. AVL Trees
6.4. Red-Black Trees
6.5. B-Trees
6.6. Tree Traversals
6.7. Applications of Trees
Chapter 7: Graphs
7.1. Introduction to Graphs
7.2. Representation of Graphs
7.3. Graph Traversals (DFS, BFS)
7.4. Shortest Path Algorithms (Dijkstra’s Algorithm)
7.5. Minimum Spanning Tree (Prim’s and Kruskal’s Algorithms)
7.6. Topological Sorting
7.7. Network Flow and Matching Algorithms
Chapter 8: Hashing
8.1. Hash Functions
8.2. Hash Tables
8.3. Collision Resolution Techniques
8.4. Open Addressing and Chaining
8.5. Hashing in Practice
8.6. Performance Analysis of Hashing
Chapter 9: Dynamic Programming and Greedy Algorithms
9.1. Introduction to Dynamic Programming
9.2. The Knapsack Problem
9.3. Matrix Chain Multiplication
9.4. Longest Common Subsequence
9.5. Optimal Substructure and Overlapping Subproblems
9.6. Greedy Algorithms
9.7. Examples of Greedy Algorithms
Chapter 10: NP-Completeness
10.1. Introduction to NP-Completeness
10.2. P, NP, and NP-Complete Problems
10.3. Reductions and the Cook-Levin Theorem
10.4. Approximations for NP-Complete Problems
10.5. Famous NP-Complete Problems
10.6. Strategies for Dealing with NP-Completeness
Chapter 11: Advanced Data Structures
11.1. Skip Lists
11.2. Heaps and Priority Queues
11.3. Tries
11.4. Disjoint Set Data Structures
11.5. Suffix Trees and Arrays
11.6. Advanced Tree Structures
Chapter 12: Algorithm Design Techniques
12.1. Divide and Conquer
12.2. Greedy Strategy
12.3. Dynamic Programming
12.4. Backtracking
12.5. Branch and Bound
12.6. Randomized Algorithms
Chapter 13: Application of Data Structures and Algorithms
13.1. String Matching Algorithms
13.2. Computational Geometry
13.3. Text Processing Algorithms
13.4. Network Flow Algorithms
13.5. Computational Biology
13.6. Real-World Applications of Algorithms
Appendices
- A. C++ Programming Language Overview
- B. Additional References and Resources
- C. Answers to Selected Problems
- D. Index
People also search for Outlines and Highlights for Data Structures and Algorithm Analysis in C++ 3rd:
outline of data
auto outline creates an outline based on the data structure
data structures outline
outline data hierarchy
a detailed outline