Computer Systems: A Programmer’s Perspective 2nd edition by Randal Bryant, David Richard O’Hallaron – Ebook PDF Instant Download/Delivery. 013034074X 978-0130340740
Full download Computer Systems: A Programmer’s Perspective 2nd edition after payment
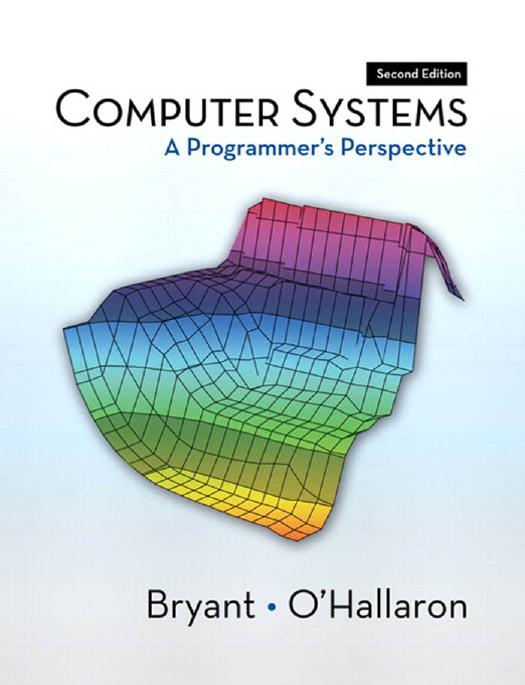
Product details:
ISBN 10: 013034074X
ISBN 13: 978-0130340740
Author: Randal Bryant, David Richard O’Hallaron
This book explains the important and enduring concepts underlying all computer systems, and shows the concrete ways that these ideas affect the correctness, performance, and utility of application programs. The book’s concrete and hands-on approach will help readers understand what is going on ?under the hood? of a computer system. This book focuses on the key concepts of basic network programming, program structure and execution, running programs on a system, and interaction and communication between programs. For anyone interested in computer organization and architecture as well as computer systems.
Computer Systems: A Programmer’s Perspective 2nd Table of contents:
Preface
- Overview of the Second Edition
- Key Changes from the First Edition
- Acknowledgments
Chapter 1: A Tour of Computer Systems
- The Layers of Computer Systems
- The Role of a Programmer in the Context of a Computer System
- The Relationship Between Hardware, Operating Systems, and Applications
- A Simple Example: Running a Program from Start to Finish
Chapter 2: Representing and Manipulating Information
- Representing Data in Computers
- Binary and Hexadecimal Number Systems
- Signed and Unsigned Integer Representation
- Floating-Point Representation
- Character Encoding (ASCII, Unicode)
- Data Type Alignment and Memory Representation
Chapter 3: Machine-Level Representation of Programs
- Translating C Programs into Machine Code
- Understanding Assembly Language
- The Structure of an Assembly Program
- The Role of the Compiler
- The Machine Instructions Set
- How a CPU Executes Instructions
- Data Movement, Arithmetic, and Control Flow in Assembly
Chapter 4: Processor Architecture
- The Basic Structure of a Processor (CPU)
- Registers, ALU, and Control Units
- The Fetch-Decode-Execute Cycle
- Interrupts and Exceptions
- Pipelines and Performance Optimizations
- CPU Memory Hierarchy: Caches, RAM, and Virtual Memory
- Instruction Set Architecture (ISA)
Chapter 5: Optimizing Program Performance
- Performance Metrics and Benchmarks
- The Role of the Compiler in Optimization
- Code Optimization Techniques: Loop Unrolling, Inlining, and More
- Cache Optimization: Data Locality and Access Patterns
- The Impact of Branch Prediction and Pipelining
- Performance Analysis Tools
Chapter 6: The Memory Hierarchy
- Memory Components: Registers, Cache, Main Memory, and Disk
- Accessing Data in Memory: Locality of Reference
- Cache Memory: Types, Design, and Usage
- Virtual Memory: Paging and Segmentation
- The Translation Lookaside Buffer (TLB)
- Memory Allocation in C: Stack vs. Heap
- Dynamic Memory Allocation and Garbage Collection
Chapter 7: Linking
- Object Files and Executables
- Static vs. Dynamic Linking
- The Linker: Responsibilities and Process
- Relocation and Symbol Resolution
- Dynamic Libraries and Shared Objects (DLLs)
- Static vs. Shared Libraries
- How the Loader Works
Chapter 8: Exceptional Control Flow
- Interrupts and System Calls
- Exception Handling Mechanisms
- The Role of the Operating System in Exception Handling
- Signals and Their Use in UNIX/Linux
- Setting Up Handlers for Signals and Exceptions
- Context Switching and Multitasking
Chapter 9: Virtual Memory
- The Concept of Virtual Memory and Its Benefits
- Paging and Page Tables
- The Translation from Virtual to Physical Address
- Page Faults and Handling Virtual Memory Exceptions
- Virtual Memory Algorithms: LRU, FIFO, and More
- The Role of the OS in Memory Management
Chapter 10: System-level I/O
- I/O Devices and Their Interface with the CPU
- The Role of Device Drivers
- Disk I/O: File System and Block Allocation
- Buffering, Caching, and Spooling
- Asynchronous vs. Synchronous I/O
- Network I/O and Sockets Programming
Chapter 11: Network Programming
- The Basics of Networking: Protocols, IP Addresses, and Ports
- Introduction to Sockets and Socket Programming
- Client-Server Model of Network Communication
- TCP vs. UDP: Reliable vs. Unreliable Communication
- Writing Network Programs: An Example of a Simple HTTP Server
- Security Considerations in Network Programming
Chapter 12: Concurrent Programming
- The Basics of Concurrent Programming
- Threads, Processes, and Synchronization
- Race Conditions and Critical Sections
- Mutexes, Semaphores, and Monitors
- Deadlock and Its Prevention
- Tools for Debugging Concurrent Programs
Chapter 13: System Security
- Basics of Computer System Security
- Buffer Overflow Attacks and Preventive Measures
- Exploiting Security Vulnerabilities in Programs
- Stack Canaries and Data Execution Prevention (DEP)
- Address Space Layout Randomization (ASLR)
- Secure Coding Practices and Tools for Preventing Security Vulnerabilities
- Cryptography and Secure Communication
Chapter 14: Conclusion: A Programmer’s Perspective on the System
- Putting It All Together: How System Understanding Makes a Better Programmer
- The Evolution of Computer Systems and the Programmer’s Role
- Future Trends in Systems and Hardware
- Final Thoughts and Further Learning Resources
Appendices
- A. C Language Reference
- B. Assembly Language Reference
- C. Unix/Linux Command Reference
- D. Glossary of Terms
- E. Index
People also search for Computer Systems: A Programmer’s Perspective 2nd:
computer security fundamentals 3rd edition pdf
computer security fundamentals 4th edition
computer security fundamentals pdf
cyber security fundamentals army quizleT
cyber security fundamentals answers